Just started with Vue? Please read part 1 first. It’s a small introduction into the Vue framework.
In part one we have created our first application using Vue. Functionally that application is complete but it is lacking a nice architecture, it is not translated, it did not have any unit tests and we did not leverage the complete power of single file components and CLI tooling to scaffold the project.
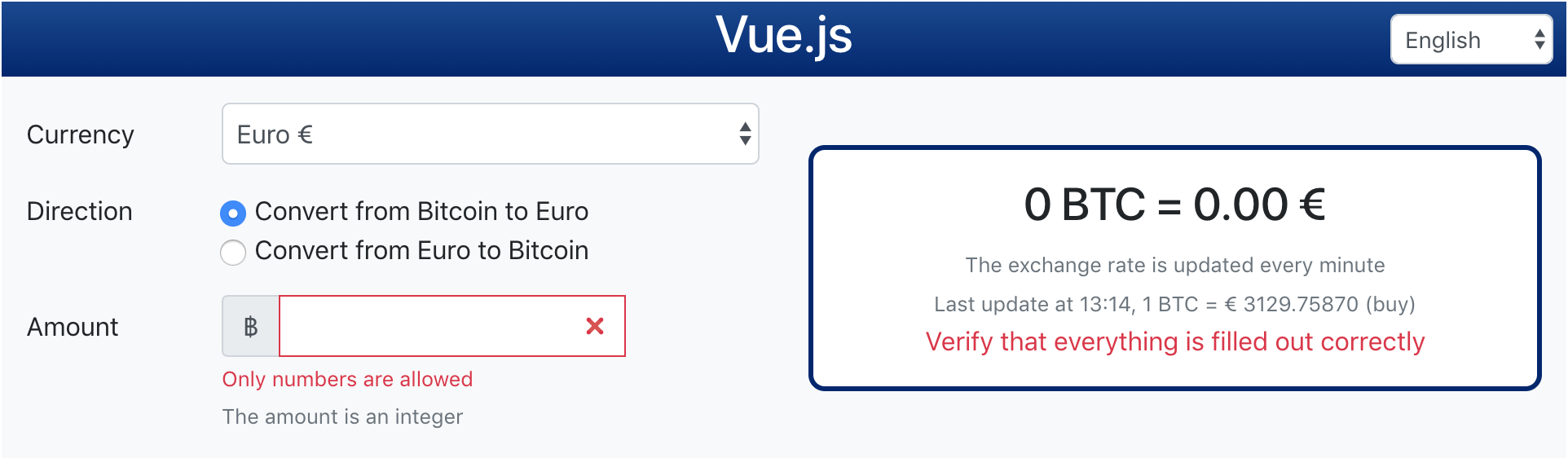
The following chapters describe in arbitrary order the additions to the Bitcoin Converter application compared to part 1…
Single-File Components
This is something I really like. Having a single file component we can put together all the code related to one component into one single file. The resulting file has the .vue
extension and includes code for the template, the script and the styling.
The benefits are that we now have complete syntax highlighting, code isolation using modules (using import) and have scoped CSS. The compilation is handled by the Webpack build tool and that means that instead of JavaScript we can use any language supported by Babel. This flexibility also applies to the styling and the template language.
An example of a single-component .vue file:
(source: Github gist)
<template> {{ greeting }} World! </template> <img src="data:image/gif;base64,R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7" data-wp-preserve="%3Cscript%3E%0Amodule.exports%20%3D%20%7B%0A%20%20data%3A%20function%20()%20%7B%0A%20%20%20%20return%20%7B%0A%20%20%20%20%20%20greeting%3A%20'Hello'%0A%20%20%20%20%7D%0A%20%20%7D%0A%7D%0A%3C%2Fscript%3E" data-mce-resize="false" data-mce-placeholder="1" class="mce-object" width="20" height="20" alt="<script>" title="<script>" /> <img src="data:image/gif;base64,R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7" data-wp-preserve="%3Cstyle%20scoped%3E%0Ap%20%7B%0A%20%20font-size%3A%202em%3B%0A%20%20text-align%3A%20center%3B%0A%7D%0A%3C%2Fstyle%3E" data-mce-resize="false" data-mce-placeholder="1" class="mce-object" width="20" height="20" alt="<style>" title="<style>" />
Vue CLI
Vue CLI is a full system for rapid Vue development. It is a runtime dependency that is built on top of Webpack. Vue CLI aims to be the standard tooling baseline for the Vue ecosystem.
Vue CLI needs to be installed globally. When installed, start a new Vue project using the command vue create <app-name>
. It asks questions about what build tool and what package management system to use and after that it creates the complete project structure.
Now you are ready to start developing the new project:
npm run serve
Vuex Datastore
Vuex is a state management pattern and library for Vue applications. It serves as a centralized store for all the components within an application.
What is a “State Management Pattern”? Actually it is the shared state of the components managed on a single location in a global singleton. With this single location for state, all the components can access and modify the state no matter where they are in the component tree.
The basic idea behind Vuex is inspired by Flux and Redux.
Although Vuex helps us deal with shared state management, it also comes with the cost of more concepts and boilerplate. It is a trade-off between short term and long term productivity.
Vue I18n
Vue I18n is internationalization plugin for Vue.js. It integrates localization features into your Vue.js Application.
When installed, using localized labels is as simple as:
<div id="app"> {{ $t("message.hello") }} </div>
Unit Testing
Last but not least; Code needs to be tested!
When using Vue CLI, we can easily add unit tests using Jest in an already created project:
vue add @vue/unit-jest
Tests are by default placed in /tests/unit
and have the .spec.js
file extension. A test might look light this:
import {createLocalVue, mount} from '@vue/test-utils' it('renders with the initial properties', () => { const Vue = createLocalVue(); const wrapper = mount(InputAmount /* .vue Component */, { localVue: Vue, propsData: { amount: 100 } }); const textInput = wrapper.find('input[type="text"]'); expect(textInput.element.value).toBe('100'); });
To start all tests:
$ vue-cli-service test:unit $ npm run test:unit (this is just an alias)
Resources
- Vue.js 2.5 – The Progressive JavaScript Framework
- Vuex 3.0 – State management pattern + library for Vue.js applications
- vee-vaidate 4.1 – Template Based Validation Framework for Vue.js
- Vue I18n 8.7 – Vue I18n is internationalization plugin for Vue.js
- Vue CLI 3 – Standard Tooling for Vue.js Development
- Bootstrap 4.2 – Responsive, mobile-first front-end component library
- Axios 0.18 – Promise based HTTP client for the browser and node.js
- Coindesk API – CoinDesk Bitcoin Price Index API
One thought on “Vue.js; AngularJS done right! – part 2”