This post describes briefly my first impressions of working with the Vue frontend framework.
I have been using Vue for only a couple of days now and during these days I have created an example application (a bitcoin converter).
Before, I have created the same application using other frameworks like AngularJS and Backbone, so this makes a comparison between the use of different frameworks possible.
What is Vue?
According to Wikipedia:
Vue (pronounced /vjuː/, like view) is a progressive framework for building user interfaces.
Wikipedia
The core library is focused on the view layer only. Vue is perfectly capable of powering sophisticated Single-Page Applications.
Components
Vue allows to build large-scale applications composed of small, self-contained and often reusable components with their own view and data logic.
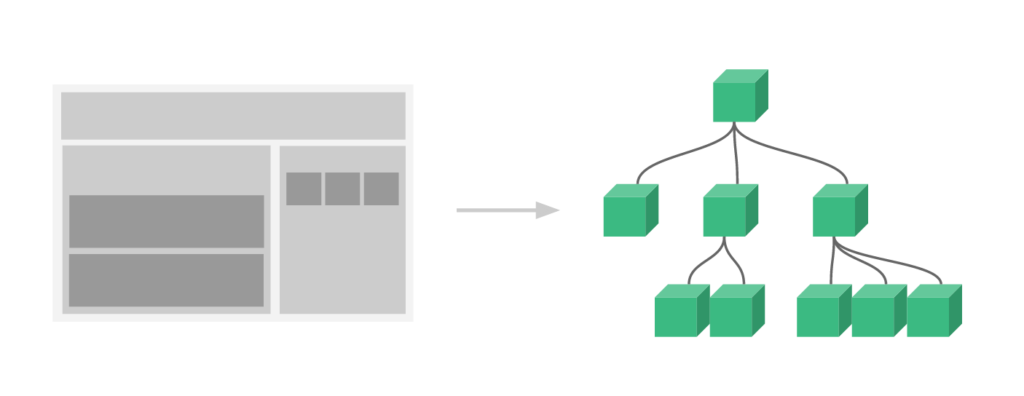
In Vue, a component is essentially a Vue instance with pre-defined options. Registering a component in Vue is straightforward:
// Define a new component called todo-item.
Vue.component('todo-item', {
template: '<li>This is a todo</li>'
})
Now you can compose it in another component’s template:
<ol>
<!-- Create an instance of the todo-item component -->
<todo-item></todo-item>
</ol>
Vue’s reactivity system
When a Vue instance is created, it adds all the properties found in its data object to Vue’s reactivity system. When the values of those properties change, the view will “react” by updating to match the new values.
I found it very similar to Angular’s scope and digest cycle with the exception that Vue does not have a separate digest cycle to handle the update of the DOM elements.
Template Syntax
Some of Vue’s syntax looks very similar to AngularJS (e.g. v-if vs ng-if). This is because there were a lot of things that AngularJS got right and these were an inspiration for Vue very early in its development.
Vue uses an HTML-based template syntax that allows you to declaratively bind the rendered DOM to the underlying Vue instance’s data. Vue can intelligently figure out the minimal number of components to re-render and apply the minimal amount of DOM manipulations when the app state changes.
<span>Message: {{ msg }}</span>
It supports the full power of JavaScript expressions inside all data bindings
{{ message.split('').reverse().join('') }}
Single File Components
A really nice concept so far only seen in Vue is the single file component.
These files have the .vue extension and contain all the code for a single component. HTML, JavaScript and CSS.
The single file concept is not yet incorporated in the demo application I mentioned in this blog post.
Documentation
Vue is without doubt the best documented frontend framework. The cookbook is really well written with lots of examples. It got me up to speed with Vue quick.
Example Application; Bitcoin Converter
The example application is a simple currency converter for Bitcoin.
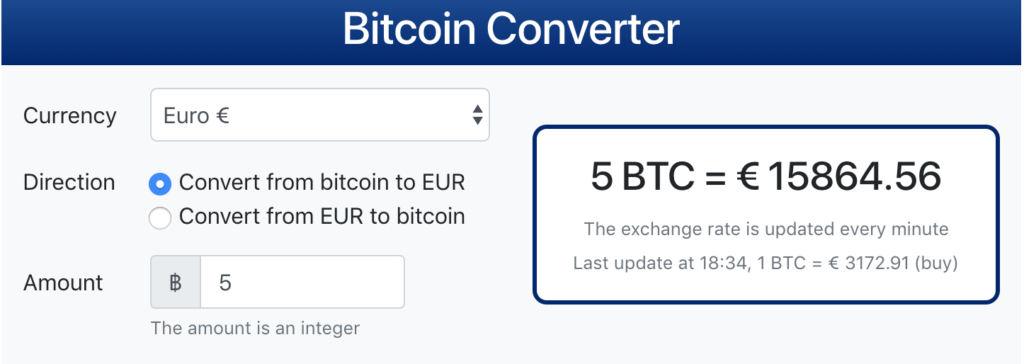
An application that contains field validation, binding of data between model and template, live update of the exchange price calculation and a call to a remote REST endpoint.
Comparison with AngularJS
Actually, working with Vue feels similar to working with the early versions of AngularJS.
The only thing lacking in Vue is the two-way binding, but that actually is a good thing. To send the updated value back to the parent, you have to explicitly program it to send the modified value as an event back to the parent.
Conclusion
This is just my first attempt to build an application using Vue. For a future version, these are the topics I still have to take a look at:
- State management with Vuex
- Single file template .vue
- TypeScript
- Services
- Transitions & Animations
- Vue CLI
Resources
- Vue.js 2.5 – The Progressive JavaScript Framework
- Bootstrap 4.2 – Responsive, mobile-first front-end component library
- Vee-validate 2.1 – Template Based Validation Framework for Vue.js
- CoinDesk Bitcoin Price Index API
Thank you for this article Rob, really interesting and clear. I’ve chosen to study Vue and interested in your comparisons to AngularJS as I’m aware of how different Angular is to AngularJS. I’ve had no experience with the former. Thanks Rob