The biggest notable new features for Vue 3 are the Composition API and full support for TypeScript. Overall Vue 3 is mostly backwards compatible. The only change I’ve found rewriting the Bitcoin Converter is that in 3.x, filters are removed and no longer supported. Instead, they can be replaced by a method call.
And there’s a new build tool in the Vue ecosystem called Vite. Its dev server is 10-100x faster than Vue CLI’s. We used Vite instead of Vue-CLI this time to scaffold the project and to start the dev server.
Composition API
To read in detail about the Composition API, see the original Vue documentation about the Composition API: An introduction to the Composition API.
Basically everything for the component is now initialised in the new setup function. This function is executed before the component is created, once the props are resolved, and serves as the entry point for composition API’s.
Reactive variables need to be wrapped in ref() functions.
A components setup function might look like this:
import { fetchUserRepositories } from '@/api/repositories' import { ref, onMounted, watch, toRefs } from 'vue' setup (props) { const { user } = toRefs(props) const repositories = ref([]) const getUserRepositories = async () => { repositories.value = await fetchUserRepositories(user.value) } onMounted(getUserRepositories) watch(user, getUserRepositories) return { repositories, getUserRepositories } }
TypeScript
TypeScript enables us to use types and have those types checked during the compile phase.
setup(props: Readonly<IProps>) { const loadingStatus = ref<String>(LOADING_STATUS.NOT_LOADING); const loadingError = ref<String>(); const tickerPrice = ref<TickerPrice>(); }
“Vite” Build Tooling
Similar to Vue CLI, Vite is also a build tool providing basic project scaffolding and a dev server.
However, Vite is not based on Webpack and has its own dev server which utilizes native ES modules in the browser. This architecture allows is to be orders of magnitude faster than Webpack’s dev server.
Vite is currently in beta and it appears that the aim of the Vite project is not to be an all-in-one tool like Vue CLI, but to focus on providing a fast dev server and basic build tool.
Demo
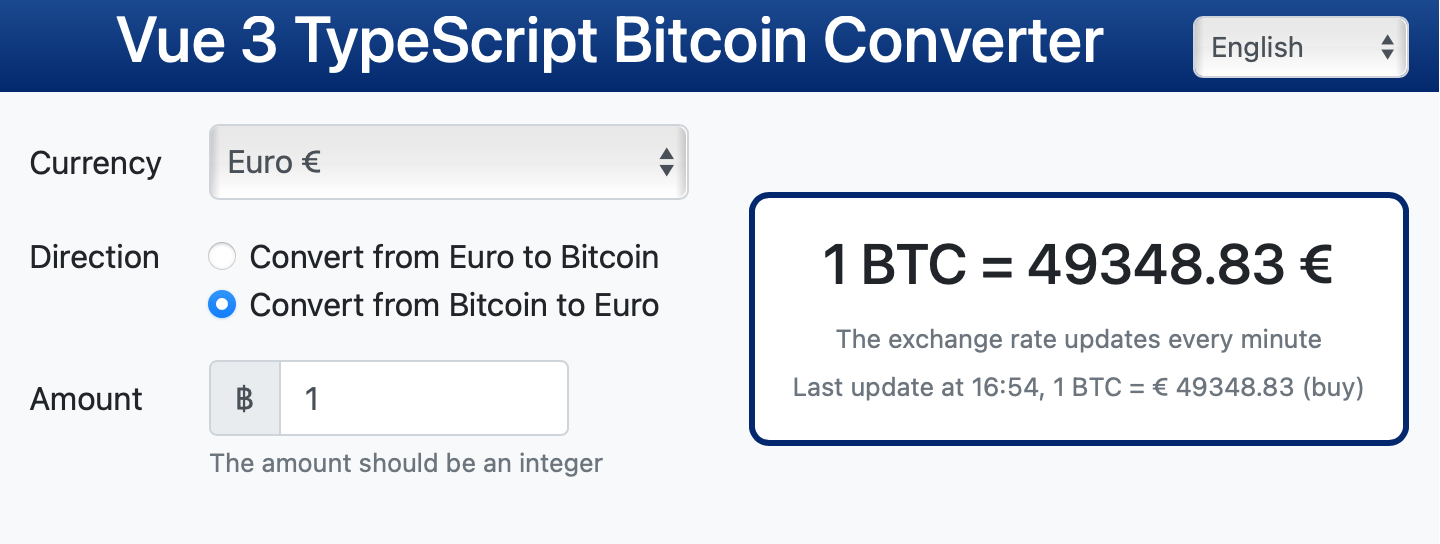
https://sandbox.juurlink.org/vue3/
Resources
- Vue.js 3.0 – The Progressive JavaScript Framework
- Vue Vite – Next Generation Frontend Tooling
- Has Vite Made Vue CLI Obsolete?
- Vue I18n 9.0 – Vue I18n is internationalization plugin for Vue.js
- Bootstrap 4.6 – Responsive, mobile-first front-end component library
- Axios 0.21 – Promise based HTTP client for the browser and node.js
- Coindesk API – CoinDesk Bitcoin Price Index API
All variants of the Bitcoin Converter Application
- 2021 – Vue 3 TypeScript Bitcoin converter application blog src demo
- 2020 – Vue.js Bitcoin converter application – part 2 blog src demo
- 2019 – Vue.js Bitcoin converter application – part 1 blog src demo
- 2017 – Angular Bitcoin converter application [blog] src demo
- 2013 – AngularJS Bitcoin converter application blog src demo
- 2013 – Backbone Bitcoin converter application blog src demo